Examples
This topic provides some examples of how to create queries and mutations for the Shopify API. In all the examples, we connect to the Shopify Admin API. Our store is called altovamapforcetest.
For instructions on how to connect to the Shopify API, see the topic Shopify API and GraphQL API. For information about how to work with the editor in the GraphQL Settings dialog, see the topic Query/Mutation Editor.
Example 1: Retrieve the first 10 products
In this example, our goal is to retrieve the details of the first 10 products from our shop. The GraphQL Settings dialog below shows the URL of our store and the access token as a header parameter as well as the definition of a query operation.
The query operation has been defined as follows:
•The root operation type is the query object type - it serves as an entry point to the API.
•The query structure specifies the selection set of fields we are interested in. In our example, we would like to retrieve a list of products.
•The products field has a first : 10 argument that limits the number of retrieved products to the first 10 items.
•The nodes field is a connection field that is used to access individual product data from the products list.
•The id and title fields specify the product attributes to return.
Query definition shown in GIF
For a quick demo showing how to create a query in the editor of the GraphQL Settings dialog, see the GIF in the topic Query/Mutation Editor.
Mapping
As soon as you click OK in the GraphQL Settings dialog, a web service call component appears in the mapping area. The response structure in the component is determined by the Shopify GraphQL schema and the fields selected in the query (in our example, id and title of the first 10 products). The response is mapped to a CSV file (screenshot below).
Output
The following response has been retrieved from the server:
gid://shopify/Product/9881539871060,Very Nice Gift Card
gid://shopify/Product/9881539903828,Cool Snowboard
gid://shopify/Product/9881539936596,Super Snowboard
gid://shopify/Product/9881539969364,Epic Ski Wax
gid://shopify/Product/9881540002132,Super-Duper Snowboard
gid://shopify/Product/9881540034900,Crazy Snowboard
gid://shopify/Product/9881540067668,Best Snowboard
gid://shopify/Product/9881540100436,Incredible Snowboard
gid://shopify/Product/9881540133204,Fantastic Snowboard
gid://shopify/Product/9881540165972,Terrific Snowboard
Example 2: Retrieve details of a particular product
In this example, our goal is to retrieve the details of a product with a particular ID. The query operation (screenshot below) has been defined as follows:
•The query uses a variable called $productId. The variable is of type ID!. The ! mark indicates that input is required. An input value will be supplied to the Request part of the web service call component.
•The product field will retrieve a single product from Shopify, using the product's productId.
•The variants field retrieves product variations (in terms of size, color, etc.).
•The variants field has a first : 10 argument, which means only the first 10 variants will be retrieved.
•Inside the variants sub-tree, we request data about each variant by selecting the following fields inside the nodes field: displayName, price, updatedAt, availableForSale, and taxable.
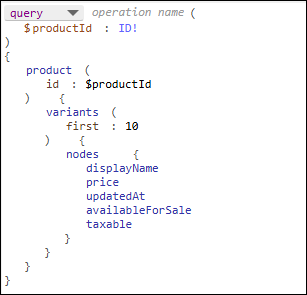
Mapping
The mapping with the configured web service call is shown below. The Request part of the call contains the input parameter (productId) we previously defined in the query operation. This input parameter receives a product's ID from the constant. The response structure is mapped to a JSON file.
Output
The following data has been retrieved from the server:
[
{
"displayName": "Legendary Snowboard",
"price": "749.95",
"updatedAt": "2024-10-29T19:55:34Z",
"availableForSale": true,
"taxable": true
}
]
Example 3: Create a new product
In this example, our goal is to create a new product on the server. For this purpose, we have defined a mutation (screenshot below) as follows:
•The mutation has a variable called $product that contains input data for the productCreate mutation function. The variable will enable us to supply the product details dynamically - to the Request part of the web service call. The variable is of type ProductCreateInput!, which is a predefined data type in the Shopify GraphQL schema. The ! mark indicates that input is required.
•The productCreate mutation function creates a new product.
•The productCreate mutation function has a product argument that accepts the $product variable as its value.
•The product sub-tree defines the fields we want Shopify to return after the new product has been created. These fields include: id, title, and descriptionHtml.
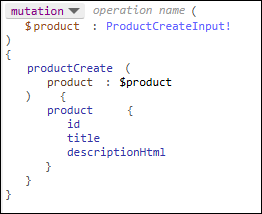
Mapping
The mapping with the configured web service call is illustrated below. Since the id field is auto-generated, we have supplied values only for the title and descriptionHtml fields. Note that the data you submit for a new product does not have to coincide with the selected fields in the Response structure: For example, you can supply several product details to the Request structure (e.g., titles, prices, variants, etc.) but retrieve only a subset of this data (e.g., only titles).
The response is mapped to a JSON file.
Output
The following data has been retrieved from the server:
[
{
"productCreate": {
"product": {
"id": "gid://shopify/Product/11863012147540",
"title": "Awesome Snowboard",
"descriptionHtml": "<p>This awesome snowboard is for the bold rider who demands power and precision. Choose the color and size that fits your style and your adventure.</p>"
}
}
}
]
Example 4: Retrieve the full response (debugging)
According to the GraphQL Specification, the data returned by the query or mutation resides in the data key. Besides the data key, there is also an errors key and an extensions key. The errors key contains any errors that occur during execution. The extensions key provides additional service-specific data, usually for debugging and logging purposes.
For testing and debugging purposes, you may want to map the full response. The easiest way to do this would be to map the response object containing the values of the data, errors, and extensions keys to an any JSON schema. The main idea is that any is a flexible data type that can match any valid JSON data. This also means that you do not have to create a special schema corresponding to the Response structure.
To define such a JSON schema, create a JSON schema file (for example, any.schema.json), open the JSON schema in an editor, and type the value {}.
The mapping below illustrates a web service call whose response is mapped to an any JSON schema.
Output
Besides IDs and product names, the following data has been retrieved from the server:
"extensions": {
"cost": {
"requestedQueryCost": 6,
"actualQueryCost": 6,
"throttleStatus": {
"maximumAvailable": 2000,
"currentlyAvailable": 1994,
"restoreRate": 100
}
}
}