Variables
Any non-trivial SPL file will require variables. Some variables are predefined by the code generator, and new variables may be created simply by assigning values to them.
The $ character is used when declaring or using a variable, a variable name is always prefixed by $. Variable names are case sensitive.
Variables types:
•integer - also used as boolean, where 0 is false and everything else is true
•string
•object - provided by UModel
•iterator - see foreach statement
Variable types are declared by first assignment:
[$x = 0] |
x is now an integer.
[$x = "teststring"] |
x is now treated as a string.
Strings
String constants are always enclosed in double quotes, like in the example above. \n and \t inside double quotes are interpreted as newline and tab, \" is a literal double quote, and \\ is a backslash. String constants can also span multiple lines.
String concatenation uses the & character:
[$BasePath = $outputpath & "/" & $JavaPackageDir] |
Objects
Objects represent the information contained in the UModel project. Objects have properties, which can be accessed using the . operator. It is not possible to create new objects in SPL (they are predefined by the code generator, derived from the input), but it is possible to assign objects to variables.
Example:
class [=$class.Name] |
This example outputs the word "class", followed by a space and the value of the Name property of the $class object.
The following table shows the relationship between UML elements their SPL equivalents along with a short description.
Predefined variables
UML element | SPL property | Multiplicity | UML Attribute / | UModel Attribute / Association | Description |
---|---|---|---|---|---|
BehavioralFeature | isAbstract | isAbstract:Boolean | |||
BehavioralFeature | raisedException | * | raisedException:Type | ||
BehavioralFeature | ownedParameter | * | ownedParameter:Parameter | ||
BehavioredClassifier | interfaceRealization | * | interfaceRealization:InterfaceRealization | ||
Class | ownedOperation | * | ownedOperation:Operation | ||
Class | nestedClassifier | * | nestedClassifier:Classifier | ||
Classifier | namespace | * | namespace:Package | packages with code language <<namespace>> set | |
Classifier | rootNamespace | * | project root namespace:String | VB only - root namespace | |
Classifier | generalization | * | generalization:Generalization | ||
Classifier | isAbstract | isAbstract:Boolean | |||
ClassifierTemplateParameter | constrainingClassifier | * | constrainingClassifier | ||
Comment | body | body:String | |||
DataType | ownedAttribute | * | ownedAttribute:Property | ||
DataType | ownedOperation | * | ownedOperation:Operation | ||
Element | kind | kind:String | |||
Element | owner | 0..1 | owner:Element | ||
Element | appliedStereotype | * | appliedStereotype:StereotypeApplication | applied stereotypes | |
Element | ownedComment | * | ownedComment:Comment | ||
ElementImport | importedElement | 1 | importedElement:PackageableElement | ||
Enumeration | ownedLiteral | * | ownedLiteral:EnumerationLiteral | ||
Enumeration | nestedClassifier | * | nestedClassifier::Classifier | ||
Enumeration | interfaceRealization | * | interfaceRealization:Interface | ||
EnumerationLiteral | ownedAttribute | * | ownedAttribute:Property | ||
EnumerationLiteral | ownedOperation | * | ownedOperation:Operation | ||
EnumerationLiteral | nestedClassifier | * | nestedClassifier:Classifier | ||
Feature | isStatic | isStatic:Boolean | |||
Generalization | general | 1 | general:Classifier | ||
Interface | ownedAttribute | * | ownedAttribute:Property | ||
Interface | ownedOperation | * | ownedOperation:Operation | ||
Interface | nestedClassifier | * | nestedClassifier:Classifier | ||
InterfaceRealization | contract | 1 | contract:Interface | ||
MultiplicityElement | lowerValue | 0..1 | lowerValue:ValueSpecification | ||
MultiplicityElement | upperValue | 0..1 | upperValue:ValueSpecification | ||
NamedElement | name | name:String | |||
NamedElement | visibility | visibility:VisibilityKind | |||
NamedElement | isPublic | isPublic:Boolean | visibility <public> | ||
NamedElement | isProtected | isProtected:Boolean | visibility <protected> | ||
NamedElement | isPrivate | isPrivate:Boolean | visibility <private> | ||
NamedElement | isPackage | isPackage:Boolean | visibility <package> | ||
NamedElement | namespacePrefix | namespacePrefix:String | XSD only - namespace prefix when exists | ||
NamedElement | parseableName | parseableName:String | CSharp, VB only - name with escaped keywords (@) | ||
Namespace | elementImport | * | elementImport:ElementImport | ||
Operation | ownedReturnParameter | 0..1 | ownedReturnParameter:Parameter | parameter with direction return set | |
Operation | type | 0..1 | type | type of parameter with direction return set | |
Operation | ownedOperationParameter | * | ownedOperationParameter:Parameter | all parameters excluding parameter with direction return set | |
Operation | implementedInterface | 1 | implementedInterface:Interface | CSharp only - the implemented interface | |
Operation | ownedOperationImplementations | * | implementedOperation:OperationImplementation | VB only - the implemented interfaces/operations | |
OperationImplementation | implementedOperationOwner | 1 | implementedOperationOwner:Interface | interface implemented by the operation | |
OperationImplementation | implementedOperationName | name:String | name of the implemented operation | ||
OperationImplementation | implementedOperationParseableName | parseableName:String | name of the implemented operation with escaped keywords | ||
Package | namespace | * | namespace:Package | packages with code language <<namespace>> set | |
PackageableElement | owningPackage | 0..1 | owningPackage | set if owner is a package | |
PackageableElement | owningNamespacePackage | 0..1 | owningNamespacePackage:Package | owning package with code language <<namespace>> set | |
Parameter | direction | direction:ParameterDirectionKind | |||
Parameter | isIn | isIn:Boolean | direction <in> | ||
Parameter | isInOut | isInOut:Boolean | direction <inout> | ||
Parameter | isOut | isOut:Boolean | direction <out> | ||
Parameter | isReturn | isReturn:Boolean | direction <return> | ||
Parameter | isVarArgList | isVarArgList:Boolean | true if parameter is a variable argument list | ||
Parameter | defaultValue | 0..1 | defaultValue:ValueSpecification | ||
Property | defaultValue | 0..1 | defaultValue:ValueSpecification | ||
RedefinableElement | isLeaf | isLeaf:Boolean | |||
Slot | name | name:String | name of the defining feature | ||
Slot | values | * | value:ValueSpecification | ||
Slot | value | value:String | value of the first value specification | ||
StereotypeApplication | name | name:String | name of applied stereotype | ||
StereotypeApplication | taggedValue | * | taggedValue:Slot | first slot of the instance specification | |
StructuralFeature | isReadOnly | isReadOnly | |||
StructuredClassifier | ownedAttribute | * | ownedAttribute:Property | ||
TemplateBinding | signature | 1 | signature:TemplateSignature | ||
TemplateBinding | parameterSubstitution | * | parameterSubstitution:TemplateParameterSubstitution | ||
TemplateParameter | paramDefault | paramDefault:String | template parameter default value | ||
TemplateParameter | ownedParameteredElement | 1 | ownedParameteredElement:ParameterableElement | ||
TemplateParameterSubstitution | parameterSubstitution | parameterSubstitution:String | Java only - code wildcard handling | ||
TemplateParameterSubstitution | parameterDimensionCount | parameterDimensionCount:Integer | code dimension count of the actual parameter | ||
TemplateParameterSubstitution | actual | 1 | OwnedActual:ParameterableElement | ||
TemplateParameterSubstitution | formal | 1 | formal:TemplateParameter | ||
TemplateSignature | template | 1 | template:TemplateableElement | ||
TemplateSignature | ownedParameter | * | ownedParameter:TemplateParameter | ||
TemplateableElement | isTemplate | isTemplate:Boolean | true if template signature set | ||
TemplateableElement | ownedTemplateSignature | 0..1 | ownedTemplateSignature:TemplateSignature | ||
TemplateableElement | templateBinding | * | templateBinding:TemplateBinding | ||
Type | typeName | * | typeName:PackageableElement | qualified code type names | |
TypedElement | type | 0..1 | type:Type | ||
TypedElement | postTypeModifier | postTypeModifier:String | postfix code modifiers | ||
ValueSpecification | value | value:String | string value of the value specification |
Adding a prefix to attributes of a class during code generation
You might need to prefix all new attributes with the "m_" characters in your project.
All new coding elements are written using the SPL templates. For example, if you open UModelSPL\C#[Java]\Default\Attribute.spl, you can change the way the name is written. Namely, you can replace
write $Property.name |
with
write "m_" & $Property.name |
It is highly recommended that you immediately update your model from code after code generation, to ensure that code and model are synchronized.
Note: | As previously mentioned, copy the SPL templates one directory higher (i.e. above the default directory to UModelSPL\C#) before modifying them. This ensures that they are not overwritten when you install a new version of UModel. Please make sure that the "user-defined override default" check box is activated in the Code from Model tab of the "Synchronization Settings" dialog box. |
SPL Templates
SPL templates can be specified per UModel project using the menu option Project | Project Settings (as shown in the screenshot below). Relative paths are also supported. Templates which are not found in the specified directory, are searched for in the local default directory.
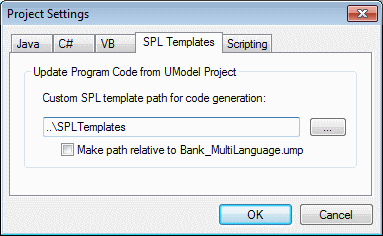
Global objects
$Options | an object holding global options: |
generateComments:bool generate doc comments (true/false) | |
$Indent | a string used to indent generated code and represent the current nesting level |
$IndentStep | a string, used to indent generated code and represent one nesting level |
$NamespacePrefix | XSD only – the target namespace prefix if present |
String manipulation routines
integer Compare(s) |
The return value indicates the lexicographic relation of the string to s (case sensitive):
<0: | the string is less than s |
0: | the string is identical to s |
>0: | the string is greater than s |
integer CompareNoCase(s) |
The return value indicates the lexicographic relation of the string to s (case insensitive):
<0: | the string is less than s |
0: | the string is identical to s |
>0: | the string is greater than s |
integer Find(s) |
Searches the string for the first match of a substring s. Returns the zero-based index of the first character of s or -1 if s is not found.
string Left(n) |
Returns the first n characters of the string.
integer Length() |
Returns the length of the string.
string MakeUpper() |
Returns a string converted to upper case.
string MakeUpper(n) |
Returns a string, with the first n characters converted to upper case.
string MakeLower() |
Returns a string converted to lower case.
string MakeLower(n) |
Returns a string, with the first n characters converted to lower case.
string Mid(n) |
Returns a string starting with the zero-based index position n
string Mid(n,m) |
Returns a string starting with the zero-based index position n and the length m
string RemoveLeft(s) |
Returns a string excluding the substring s if Left( s.Length() ) is equal to substring s.
string RemoveLeftNoCase(s) |
Returns a string excluding the substring s if Left( s.Length() ) is equal to substring s (case insensitive).
string RemoveRight(s) |
Returns a string excluding the substring s if Right( s.Length() ) is equal to substring s.
string RemoveRightNoCase(s) |
Returns a string excluding the substring s if Right( s.Length() ) is equal to substring s (case insensitive).
string Repeat(s,n) |
Returns a string containing substring s repeated n times.
string Right(n) |
Returns the last n characters of the string.